Imagine you have a magic wand that can turn lights on and off just by waving it. With Arduino, you have similar power when using a command called digital write. It lets you control things like lights, motors, and other gadgets with just a few lines of code!
Digital Write is like telling your Arduino, “Turn this on!” or “Turn this off!” You can use it to send electricity to a pin (HIGH) or stop the electricity (LOW). It’s a simple way to control almost anything connected to your Arduino.
Digital Write
Set the Pin Mode: First, tell Arduino which pin you want to control by writing pinMode(Pin, OUTPUT). This makes sure the pin is ready to send signals.
Write to the Pin: Use digitalWrite(Pin, HIGH)to turn something on (like an LED) and digitalWrite(Pin, LOW) to turn it off.
Digital Write Example: Blinking an LED
Hardware Requirements
1x Arduino UNO
1x 220R Resistor
1x Led
1x breadboard
Some jumper wire
Circuit Diagram
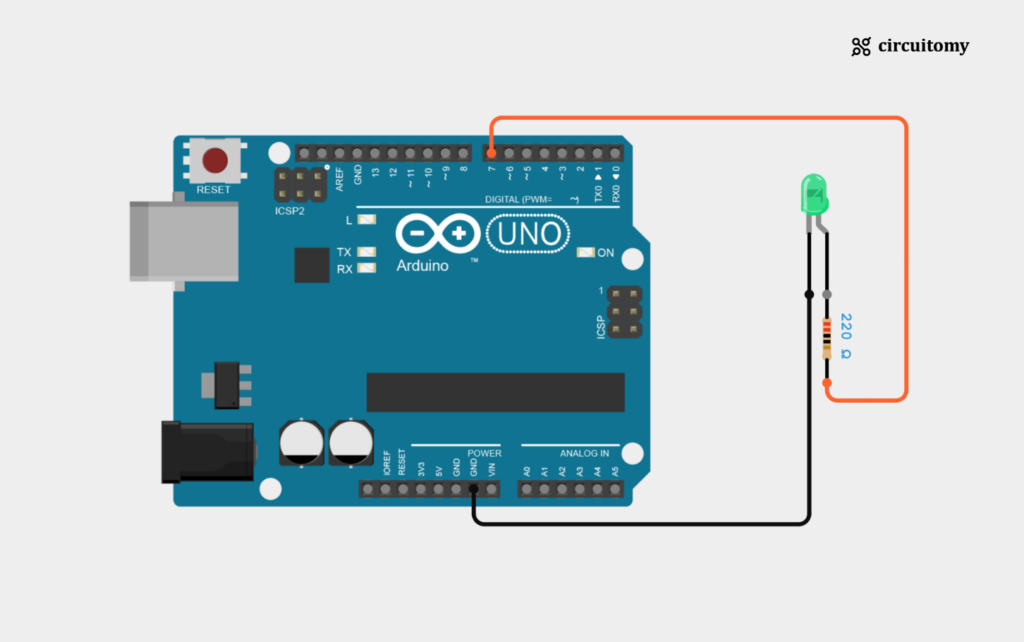
Fig: Interfacing led with Arduino
LED Connection:
LED Pin | Arduino Pin |
LED’s Positive (Anode) Pin | D7 (Digital Pin 7) |
LED’s Negative (Cathode) Pin | GND |
Code
// Set pin number
int ledPin = 7;
void setup() {
// Initialize the pin as an output
pinMode(ledPin, OUTPUT);
}
void loop() {
// Turn the LED on (HIGH is the voltage level)
digitalWrite(ledPin, HIGH);
// Wait for a second
delay(1000);
// Turn the LED off by making the voltage LOW
digitalWrite(ledPin, LOW);
// Wait for a second
delay(1000);
}
1. Set the PIN Number
int ledPin = 7;
This line defines a variable called ledPin and assigns it the value 7. This tells the Arduino that the LED is connected to pin 7.
2. Setup Function
void setup() {
// Initialize the pin as an output
pinMode(ledPin, OUTPUT);
}
The setup() function runs once when the Arduino starts. Inside this function, the pinMode()function is called to set ledPin (pin 7) as an output. This means that the pin will send out voltage to power the LED.
3. Loop Function
void loop() {
// Turn the LED on (HIGH is the voltage level)
digitalWrite(ledPin, HIGH);
// Wait for a second
delay(1000);
// Turn the LED off by making the voltage LOW
digitalWrite(ledPin, LOW);
// Wait for a second
delay(1000);
}
void loop() function runs continuously after setup(). Inside this function:
1.Turn the LED on:
digitalWrite(ledPin, HIGH);
digitalWrite(ledPin, HIGH);sets pin 7 to a HIGH voltage level (5V), which turns the LED on.
2. Wait for a second:
delay(1000);
delay(1000); pauses the program for 1000 milliseconds (1 second).
3. Turn the LED off:
digitalWrite(ledPin, LOW);
digitalWrite(ledPin, LOW); sets pin 7 to a LOW voltage level (0V), turning the LED off.
4. Wait for a second:
delay(1000);
Another 1-second pause before the loop repeats.
Application:
This code can be used in various practical applications, such as:
1. LED Indicator: It can serve as a basic status indicator (on/off) for an embedded system.
2. Timer or Notification System: The blinking LED can indicate a timed event, warning, or process completion.
3. Debugging: Blinking LEDs are often used in electronics projects to visually confirm that the program is running correctly.